In this article, we will learn about the basics of OOPs. Object-Oriented Programming is a paradigm that provides many concepts, such as inheritance, data binding, polymorphism, etc.
Object means a real-world entity such as a pen, chair, table, computer, watch, etc. Object-Oriented Programming is a methodology to design a program using classes and objects. It simplifies software development and maintenance by providing some concepts:
- Object
- Class
- Inheritance
- Polymorphism
- Abstraction
- Encapsulation
Objects
Any entity that has state and behavior is known as an object. For example, a chair, pen, table, keyboard, bike, etc. It can be physical or logical. An object contains an address and takes up some space in memory.
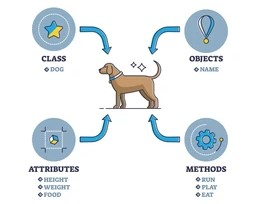
Class
Collection of objects is called class. It is a logical entity.
A class can also be defined as a blueprint from which you can create an individual object. Class doesn’t consume any space.
Object and Class Example: main within the class
//Defining a Student class.
class Student{
int id; //field or data member or instance variable
String name;
//creating main method inside the Student class
public static void main(String args[]){
//Creating an object or instance
Student s1=new Student();//creating an object of Student
//Printing values of the object
System.out.println(s1.id);//accessing member through reference variable
System.out.println(s1.name);
}
}
Here, it defines a class named Student
with two fields (id
and name
). It then creates an instance of theStudent
class (s1
) and prints the values of its fields.
The Student class contains the main method which serves as the entry point of the program. After that an object of the ‘Student‘ class named ‘s1’ has been created using the ‘new‘ keyword.
//Output
0
null
Object and class Example: main outside the class
In real time development, we create classes and use it from another class. It is a better approach than previous one. Let’s see a simple example, where we are having main() method in another class.
//Creating Student class.
class Student{
int id;
String name;
}
//Creating another class TestStudent1 which contains the main method
class TestStudent1{
public static void main(String args[]){
Student s1=new Student();
System.out.println(s1.id);
System.out.println(s1.name);
}
}
This above program defines a Student
class with two fields (id
and name
). It also creates another class named TestStudent1
, which contains the main
method. In the main
method, an object of the Student
class is created, and its fields are printed.
//Output
0
null